4 Principles of Object Oriented Programming
- megha dureja
- May 4, 2020
- 2 min read
Updated: Feb 16, 2021
There are 4 major principles that make a language Object Oriented. These are Encapsulation, Data Abstraction, Polymorphism and Inheritance. These are also called as four pillars of Object Oriented Programming.
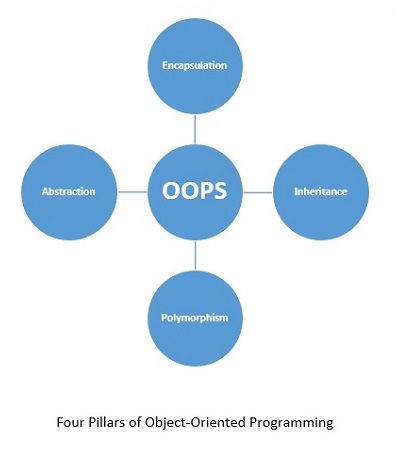
Encapsulation:-
Encapsulation is the mechanism of hiding of data implementation by restricting access to public methods. Instance variables are kept private and accessor methods are made public to achieve this.
public class Product {
private String name;
private Long id;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
}
Abstraction:-
Abstract means a concept or an Idea which is not associated with any particular instance. Using abstract class/Interface we express the intent of the class rather than the actual implementation. In a way, one class should not know the inner details of another in order to use it, just knowing the interfaces should be good enough.
// I say all motor vehicles should look like this:
interface MotorVehicle
{
void run();
int getFuel();
}
// My team mate complies and writes vehicle looking that way
class Car implements MotorVehicle
{
int fuel;
void run()
{
print("Wrroooooooom");
}
int getFuel()
{
return this.fuel;
}
}
Inheritance:-
Inheritance expresses “is-a” and/or “has-a” relationship between two objects. Using Inheritance, In derived classes we can reuse the code of existing super classes. In Java, concept of “is-a” is based on class inheritance (using extends) or interface implementation (using implements).
Example: Car is an Automobile and Car has an Engine.
class Engine {} // The Engine class
class Automobile {} // Automobile class which is parent to Car
class Car extends Automobile { // Car class extends Automobile
// Car class has an instance of Engine class as its member
private Engine engine;
}
Polymorphism:-
It means one name many forms. i.e., writing the method once and performing a number of tasks using the same method name.
It is further of two types — static and dynamic. Static polymorphism is achieved using method overloading and dynamic polymorphism using method overriding. It is closely related to inheritance. We can write a code that works on the superclass, and it will work with any subclass type as well.
class Animal{
void eat(){
System.out.println("animal is eating...");
}
}
class Dog extends Animal{
void eat(){
System.out.println("dog is eating...");
}
public static void main(String args[]){
Animal a=new Dog();
a.eat();
}
}
Kommentare