Spring Boot
- megha dureja
- May 4, 2020
- 5 min read
Updated: Feb 25, 2021
Spring Boot helps you accelerate application development.
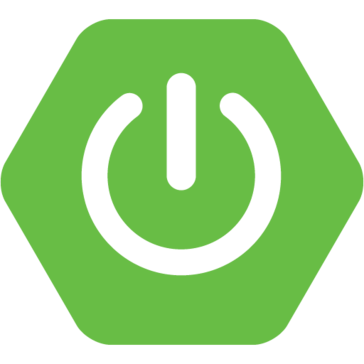
Features
1. Create stand-alone Spring applications
2. Embed Tomcat, Jetty or Undertow directly (no need to deploy WAR files)
3. Provide opinionated 'starter' dependencies to simplify your build configuration
4. Automatically configure Spring and 3rd party libraries whenever possible
5. Provide production-ready features such as metrics, health checks, and externalized configuration
6. Absolutely no code generation and no requirement for XML configuration
1) Building Web Application with Spring Boot
1. Create a maven project
2. Add below parent starter and related dependency for web
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
</dependencies>
3. Create an Application Class
@SpringBootApplication public class App { public static void main(String[] args) { SpringApplication.run(App.class, args); }
}
4. Create a simple Web Controller class
@RestController public class ResourceController { @RequestMapping("/") public String index() { return "Greetings from Spring Boot!"; } }
OR
5. Serve index.html page
By default, Spring Boot serves static content from resources in the classpath at /static (or /public). The index.html resource is special because, if it exists, it is used as a "`welcome page,"serving-web-content/ which means it is served up as the root resource (that is, at `http://localhost:8080/). As a result, you need to create the following file (which you can find in src/main/resources/static/index.html):
<!DOCTYPE HTML>
<html>
<head>
<title>Getting Started: Serving Web Content</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<p>Get your greeting <a href="/greeting">here</a></p>
</body>
</html>
6. Run the application
7. Hit the request
$ curl localhost:8080
Greetings from Spring Boot!
OR
You will see the HTML at http://localhost:8080/.
Tips:-
Disabling the Whitelabel Error Page
A WhiteLabel Error can is disabled in the application.properties file by setting the server.error.whitelabel.enabled=false
When the WhiteLabel Error Page is disabled and no custom error page is provided, the web server's error page (Tomcat, Jetty) is shown as
HTTP Status 404 – Not Found
Displaying Custom Error Message / Page
By default, Spring Boot displays a whitelabel error page if we encounter a server error. To specify the custom error logic, we need a @Controller that handles the /error path or a View that resolves with a name of error. To achieve that, we have to create an error controller bean that'll replace the default one.
@RestController
public class ResourceErrorController implements ErrorController {
@RequestMapping("/error")
public String handleError() {
//do something like logging
return "error";
}
@Override
public String getErrorPath() {
// return a custom path to call when an error occurred
return "/error";
}
}
2) Serving Web Content with Spring MVC
1. Add below dependency
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
2. Create a Web Controller
@Controller public class GreetingController { @GetMapping("/greeting") public String greeting(@RequestParam(name="name", required=false, defaultValue="World") String name, Model model) { model.addAttribute("name", name); return "greeting"; } }
@Controller :- used to create a map of model object and find a view
@RestController :- simply returns object and object data is directly written into HTTP response as JSON or XML i.e @RestController is a combination of @Controller + @ResponseBody(Spring 4). Added to make development of Restful Webservices easier.
Traditionally response from web app is generally view(html + css + javascript) and REST API just return data in form of json or xml.
@RequestMapping :- used to map web http requests(/greeting) to Spring Controller methods
@GetMapping :- shortcut for @RequestMapping(method=RequestMethod.GET)
@PathVariable :- parts of mapping URI can be bound to variables /ex/foo/{name}
@RequestParam :- binds the value of URL query string parameters /ex/foo?name=Megha
3. Create a Web Resource (greeting.html)
The following listing (from src/main/resources/templates/greeting.html) shows the greeting.html template:
<!DOCTYPE HTML> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Getting Started: Serving Web Content</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> </head> <body> <p th:text="'Hello, ' + ${name} + '!'" />
<form th:action="@{/logout}" method="post"> <input type="submit" value="Sign Out"/> </form> </body> </html>
3) Securing a Web Application by Spring Security
Setup Spring Security
Suppose that you want to prevent unauthorized users from viewing the greeting page at/greeting. So, you need to add a barrier that forces the visitor to sign in before they can see that page.
You do that by configuring Spring Security in the application. If Spring Security is on the classpath, Spring Boot automatically secures all HTTP endpoints with “basic” authentication.
1. Add below dependency
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
2. The web application is based on Spring MVC. As a result, you need to configure Spring MVC and set up view controllers to expose these templates.
@Configuration public class MvcConfig implements WebMvcConfigurer { public void addViewControllers(ViewControllerRegistry registry) { registry.addViewController("/login").setViewName("login"); } }
The addViewControllers() method (which overrides the method of the same name in WebMvcConfigurer) adds view controllers.
3. Configure web security such that only authenticated users can see the secret greeting:
@Configuration @EnableWebSecurity public class WebSecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/").permitAll() .anyRequest().authenticated() .and() .formLogin() .loginPage("/login") .permitAll() .and() .logout() .permitAll(); } @Bean @Override public UserDetailsService userDetailsService() { UserDetails user = User.withDefaultPasswordEncoder() .username("user") .password("password") .roles("USER") .build(); return new InMemoryUserDetailsManager(user); } }
The configure(HttpSecurity) method defines which URL paths should be secured and which should not.
Specifically, the / path is configured to not require any authentication. All other paths must be authenticated.
When a user successfully logs in, they are redirected to the previously requested page that required authentication.
There is a custom /login page (which is specified by loginPage()), and everyone is allowed to view it.
The userDetailsService() method sets up an in-memory user store with a single user. That user is given a user name of user, a password of password, and a role of USER.
Now you need to create the login page. There is already a view controller for the login view, so you need only to create the login view itself, as the following listing (from src/main/resources/templates/login.html) shows:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org" xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3"> <head> <title>Spring Security Example </title> </head> <body> <div th:if="${param.error}"> Invalid username and password. </div> <div th:if="${param.logout}"> You have been logged out. </div> <form th:action="@{/login}" method="post"> <div><label> User Name : <input type="text" name="username"/> </label></div> <div><label> Password: <input type="password" name="password"/> </label></div> <div><input type="submit" value="Sign In"/></div> </form> </body> </html>
This Thymeleaf template presents a form that captures a username and password and posts them to /login.
As configured, Spring Security provides a filter that intercepts that request and authenticates the user. If the user fails to authenticate, the page is redirected to /login?error, and your page displays the appropriate error message. Upon successfully signing out, your application is sent to /login?logout, and your page displays the appropriate success message.
4) Securing a Web Application by Spring Security's Embedded LDAP
1. Setup LDAP dependency
<dependency> <groupId>org.springframework.ldap</groupId> <artifactId>spring-ldap-core</artifactId> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-ldap</artifactId> </dependency> <dependency> <groupId>com.unboundid</groupId> <artifactId>unboundid-ldapsdk</artifactId> </dependency>
UnboundId is an open source implementation of LDAP server.
2. Use Java to configure your security policy.
@Configuration public class WebSecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .anyRequest().fullyAuthenticated() .and() .formLogin(); } @Override public void configure(AuthenticationManagerBuilder auth) throws Exception { auth .ldapAuthentication() .userDnPatterns("uid={0},ou=people") .groupSearchBase("ou=groups") .contextSource() .url("ldap://localhost:8389/dc=springframework,dc=org") .and() .passwordCompare() .passwordEncoder(new BCryptPasswordEncoder()) .passwordAttribute("userPassword"); } }
3. Load the LDAP server with a data file that contains a set of users.
src/main/resources/test-server.ldif
dn: dc=springframework,dc=org
objectclass: top
objectclass: domain
objectclass: extensibleObject
dc: springframework
dn: ou=groups,dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: groups
dn: ou=subgroups,ou=groups,dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: subgroups
dn: ou=people,dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: people
dn: ou=space cadets,dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: space cadets
dn: ou=\"quoted people\",dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: "quoted people"
dn: ou=otherpeople,dc=springframework,dc=org
objectclass: top
objectclass: organizationalUnit
ou: otherpeople
dn: uid=ben,ou=people,dc=springframework,dc=org
objectclass: top
objectclass: person
objectclass: organizationalPerson
objectclass: inetOrgPerson
cn: Ben Alex
sn: Alex
uid: ben
userPassword: $2a$10$c6bSeWPhg06xB1lvmaWNNe4NROmZiSpYhlocU/98HNr2MhIOiSt36
dn: cn=developers,ou=groups,dc=springframework,dc=org
objectclass: top
objectclass: groupOfUniqueNames
cn: developers
ou: developer
uniqueMember: uid=ben,ou=people,dc=springframework,dc=org
uniqueMember: uid=bob,ou=people,dc=springframework,dc=org
dn: cn=managers,ou=groups,dc=springframework,dc=org
objectclass: top
objectclass: groupOfUniqueNames
cn: managers
ou: manager
uniqueMember: uid=ben,ou=people,dc=springframework,dc=org
uniqueMember: cn=mouse\, jerry,ou=people,dc=springframework,dc=org
dn: cn=submanagers,ou=subgroups,ou=groups,dc=springframework,dc=org
objectclass: top
objectclass: groupOfUniqueNames
cn: submanagers
ou: submanager
uniqueMember: uid=ben,ou=people,dc=springframework,dc=org
4. Configure below properties in application.properties file
spring.ldap.embedded.port=8389 spring.ldap.embedded.ldif=classpath:test-server.ldif spring.ldap.embedded.base-dn=dc=springframework,dc=org
5. Visit the site at http://localhost:8080, you should be redirected to a login page provided by Spring Security. Enter a user name of ben and a password of benspassword.
Comments