Scala
- megha dureja
- Nov 20, 2020
- 3 min read
Updated: Feb 25, 2021
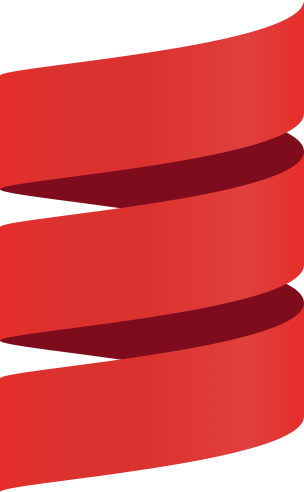
Scala is a high-level programming language that has both functional and object-oriented language features. Scala is compiled to Java bytecode. The executable then runs on a Java Virtual Machine (JVM).
Hello World in Java:
public class HelloJava {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
Hello World in Scala:
object HelloScala {
def main(args: Array[String]): Unit = {
println("Hello World!")
}
}
Scala String:-
A string is a sequence of characters. In Scala, objects of String are immutable which means a constant and cannot be changed once created.
You can create a string variable
var str = "Hello World!"
or
val str = "Hello World!"
OR
var str: String = "Hello World!"
or
val str: String = "Hello World!"
// Scala program to illustrate how to create a string
object Main
{
// str1 and str2 are two different strings
var str1 = "hello "
val str2: String = "world!"
def main(args: Array[String])
{
// Display both strings
println(str1);
println(str2);
}
}
//output
hello
world!
But because Scala offers the magic of implicit conversions, String instances also have access to all the methods of the StringOps class. You can iterate over every character in the string using the foreach method:
str1.foreach(println)
h
e
l
l
o
for (c <- str1) println(c)
h
e
l
l
o
for/yield approach:
scala> val upper = for (c <- "hello, world") yield c.toUpper
upper: String = HELLO, WORLD
Adding yield to a for loop essentially places the result from each loop iteration into a temporary holding area. When the loop completes, all of the elements in the holding area are returned as a single collection.
filter method:
str1.filter(_ != 'l')
heo
drop and take methods:
"scala".drop(2).take(2)
al
split and map methods:
val s = "eggs, milk, butter, Coco Puffs"
s.split(",").map(_.trim)
scala> val upper = "hello, world".map(c => c.toUpper)
upper: String = HELLO, WORLD
you can shorten that code using the magic of Scala’s underscore character:
scala> val upper = "hello, world".map(_.toUpper)
upper: String = HELLO, WORLD
compare two scala strings with the == operator
val s1 = "Hello"
val s2 = "Hello"
val result = s1 == s2 //scala
println(result); //true
val result = s1.equalsIgnoreCase(s2) //use java equalsIgnoreCase method
println(result); //true
In Scala, the == method defined in the AnyRef class first checks for null values, and then
calls the equals method on the first object (i.e., this) to see if the two objects are equal.
String interpolation
val name = "Fred"
val age = 33
scala> println(s"$name is $age years old.")
Fred is 33 years old.
scala> println(s"Age next year: ${age + 1}")
Age next year: 34
scala> println(s"You are 33 years old: ${age == 33}")
You are 33 years old: true
You’ll also need to use curly braces when printing object fields. The following example shows the correct approach:
scala> case class Student(name: String, score: Int)
defined class Student
scala> val hannah = Student("Hannah", 95)
hannah: Student = Student(Hannah,95)
scala> println(s"${hannah.name} has a score of ${hannah.score}")
Hannah has a score of 95
Interpolator
s, f, raw
Create your own interpolator
Java approach :-
String s = "Hello";
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
// do something with the character ...
// sb.append ...
}
String result = sb.toString();
Regex object
- by invoking the .r method on a String
- import the Regex class, create a Regex instance
Scala Numbers:-
In Scala, all the numeric types are objects, including Byte, Char, Double, Float, Int,
Long, and Short. These seven numeric types extend the AnyVal trait, as do the Unit and
Boolean classes, which are considered to be “nonnumeric value types.”
Parsing a Number from a String
Use the to* methods that are available on a String (courtesy of the StringLike trait)
scala> "100".toInt
res0: Int = 100
Scala Constructors:-
are used to initializing the object’s state. Like methods, a constructor also contains a collection of statements(i.e. instructions) that are executed at the time of Object creation.
Scala supports two types of constructors:
Primary Constructor:-
When Scala program contains only one constructor, then that constructor is known as a primary constructor. The primary constructor and the class share the same body, means we need not to create a constructor explicitly.
class class_name(Parameter_list){
// Statements...
}
In the above syntax, the primary constructor and the class share the same body so, anything defined in the body of the class except method declaration is the part of the primary constructor.
class Person(var firstName: String, var lastName: String) {
println("the constructor begins")
// some class fields
private val HOME = System.getProperty("user.home")
var age = 0
// some methods
override def toString = s"$firstName $lastName is $age years old"
def display { println("First Name: " + firstName); }
def printHome { println(s"HOME = $HOME") }
def printFullName { println(this) } // uses toString
printHome
printFullName
println("still in the constructor")
}
object Main
{
def main(args: Array[String])
{
// Creating and initialzing
val p = new Person("Adam", "Meyer")
obj.display();
}
}
//
the constructor begins
HOME = /Users/Al
Adam Meyer is 0 years old
still in the constructor
First Name: Adam
Auxiliary Constructor:-
Comments